A quick way to generate Go tests in Visual Studio Code
Click here to generate unit tests.
I just learned of a quick way to write Go tests in Visual Studio Code (VSC). While programming with a colleague, I noticed he generated a test's boilerplate code after right-clicking somewhere in the editor. That got my attention since it was my first time seeing that magic. So, I went to VSC, read a bit of documentation, and found out how he did it. In this short piece, I'll describe the process.
The tool that creates this boilerplate code is called gotests
. It generates Go's table-driven tests that cover the functions present in a Go file or generates them from a method's signature. To use it, you could either install it and execute it from the command line or directly from VSC since it is included in VSC's Go plugin. Let's see how.
Suppose you have a Go source file like this:
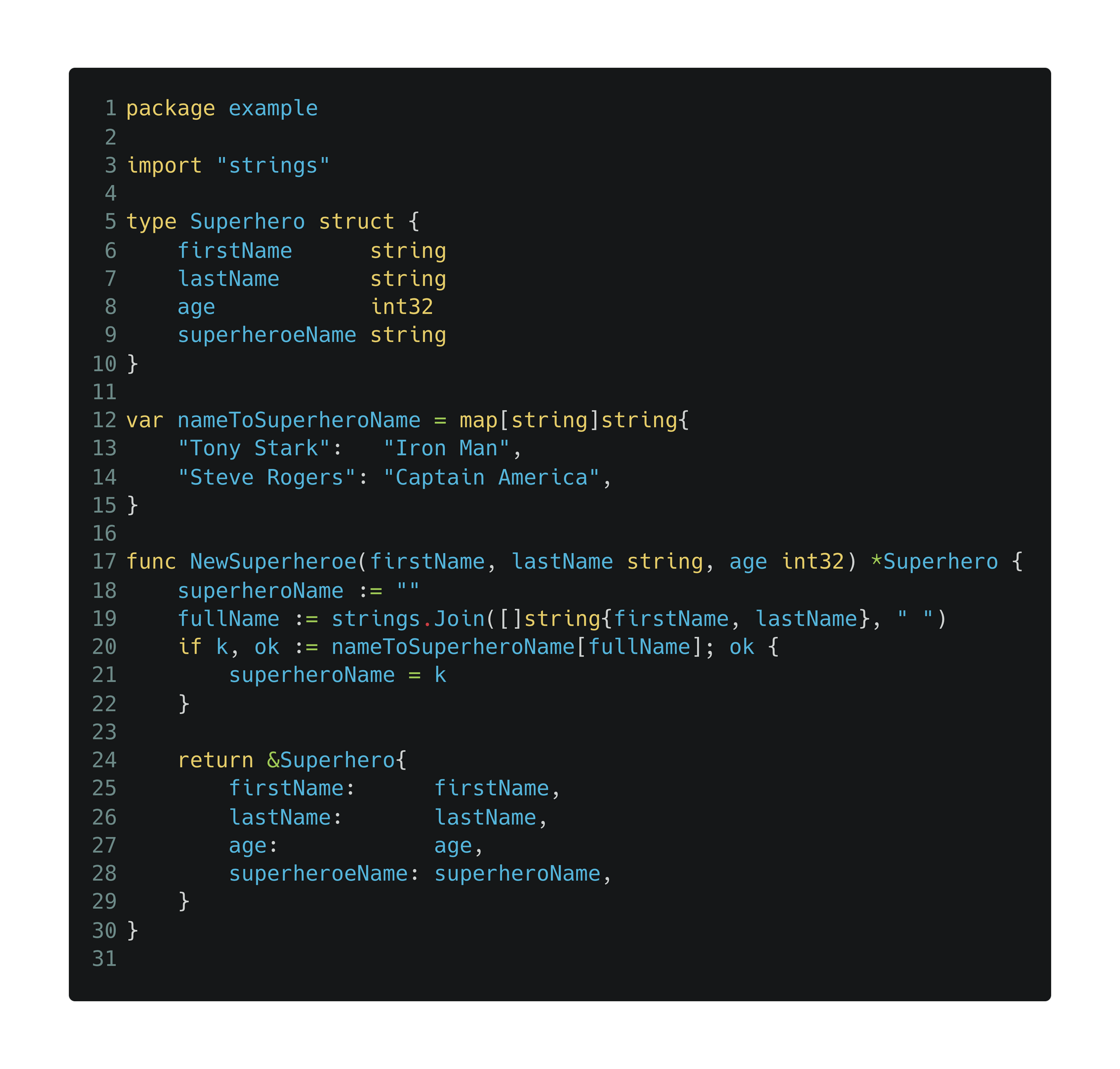
It has a struct Superhero
, a map nameToSuperheroName
to translate a superhero's real name to its alias, and a function that creates a new Superhero
and checks if the map contains its alias. To generate its boilerplate test code, right-click on the function's signature (where it says NewSuperhero
) and select the "Go: Generate Unit Tests For Function."
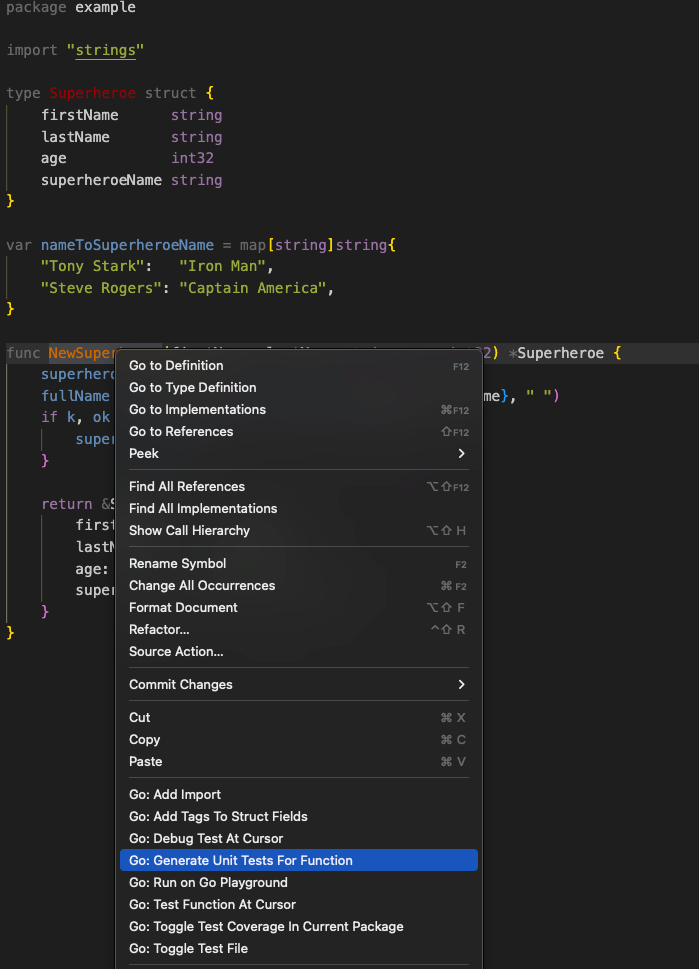
Clicking here will create a test file containing a test function named TestNewSuperheroe
and the skeleton code you need to start writing your tests. This test code follows the table-driven test pattern, a type of testing involving a struct (the "table") whose fields include the tests' inputs, expected output, and other information such as its name.
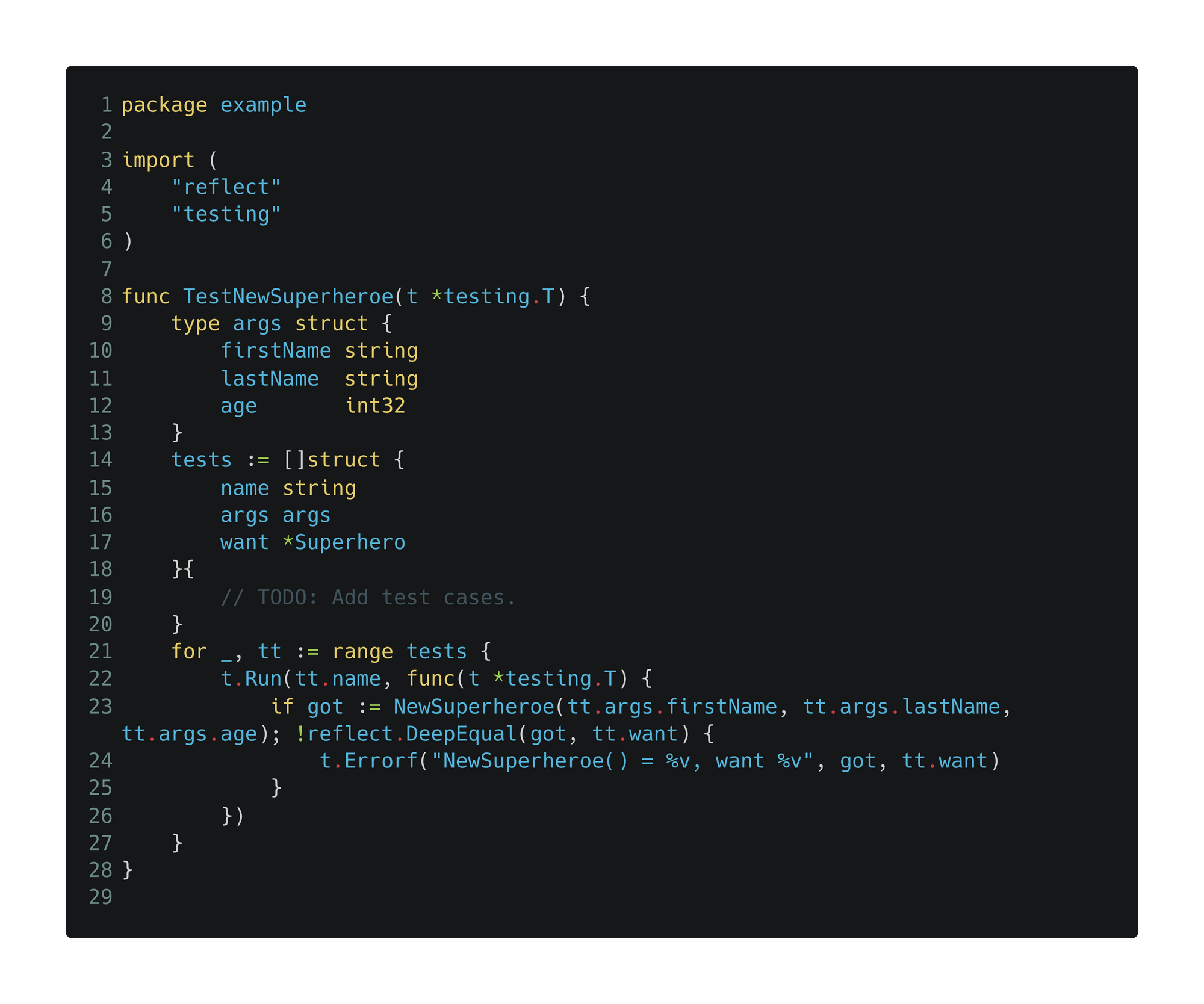
To fill out the table, you need to create a list of the anonymous struct in line 19. This struct consists of a name
string
, for assigning a name to the test, an args struct
made of the arguments of the NewSuperheroe
function, and want
, the Superhero
object you expect NewSuperhero
to output. For example, the screenshot below has my test, which I namedTest Tony Stark
. I'll use this test to verify if the inputs Tony
, Stark
, and 48
produce a Superhero
object that looks as follows:
&Superhero{
firstName: "Tony",
lastName: "Stark",
age: 48,
superheroeName: "Iron Man",
}
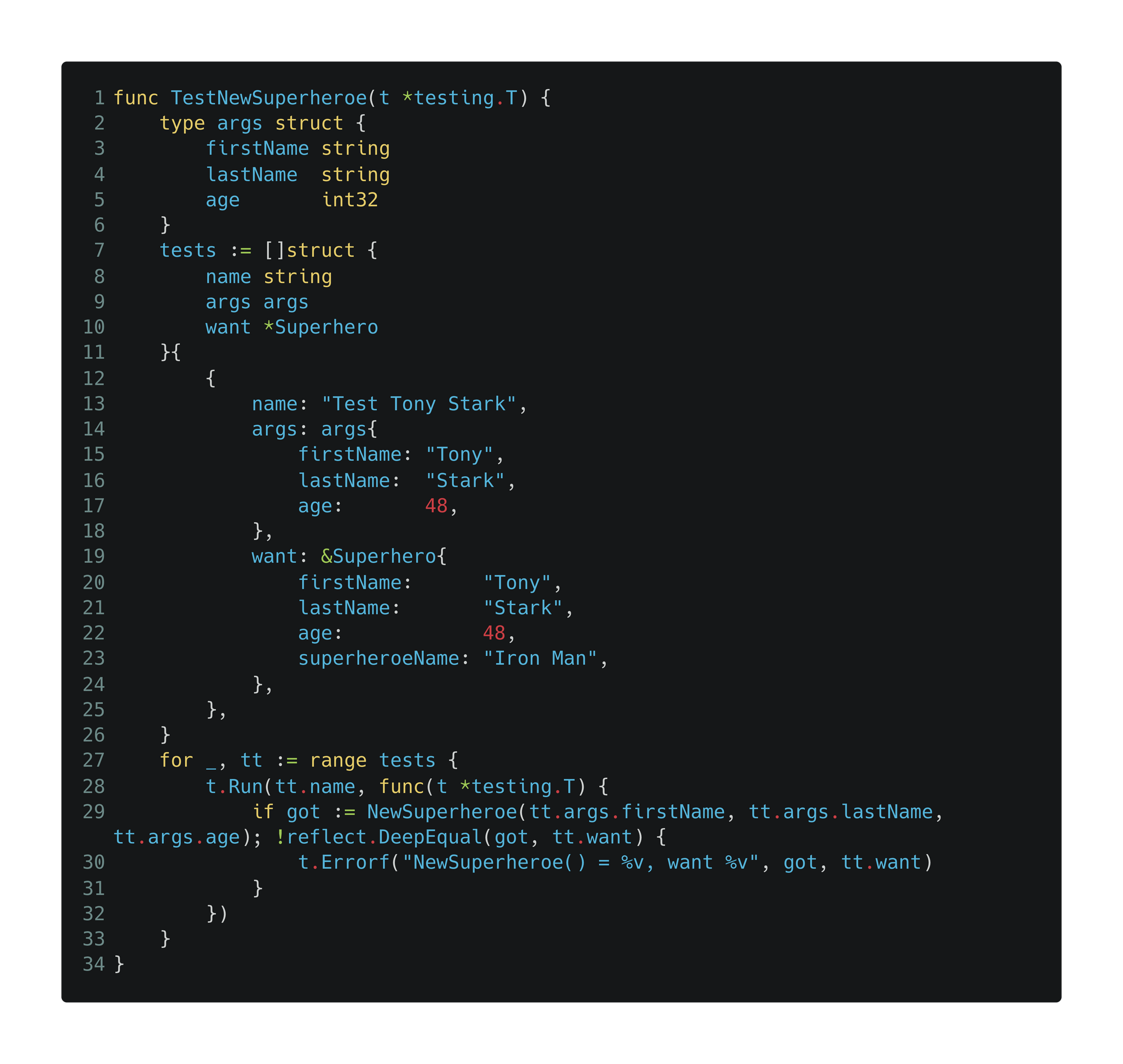
Note that the field superheroeName
is Iron Man
because Tony Stark's name translates to Iron Man according to the map defined in the source file.
But what if we want to generate tests for all the functions a file might have? For example, suppose you add two new methods, isAdult
and isAvenger
to our Superheroe
struct:
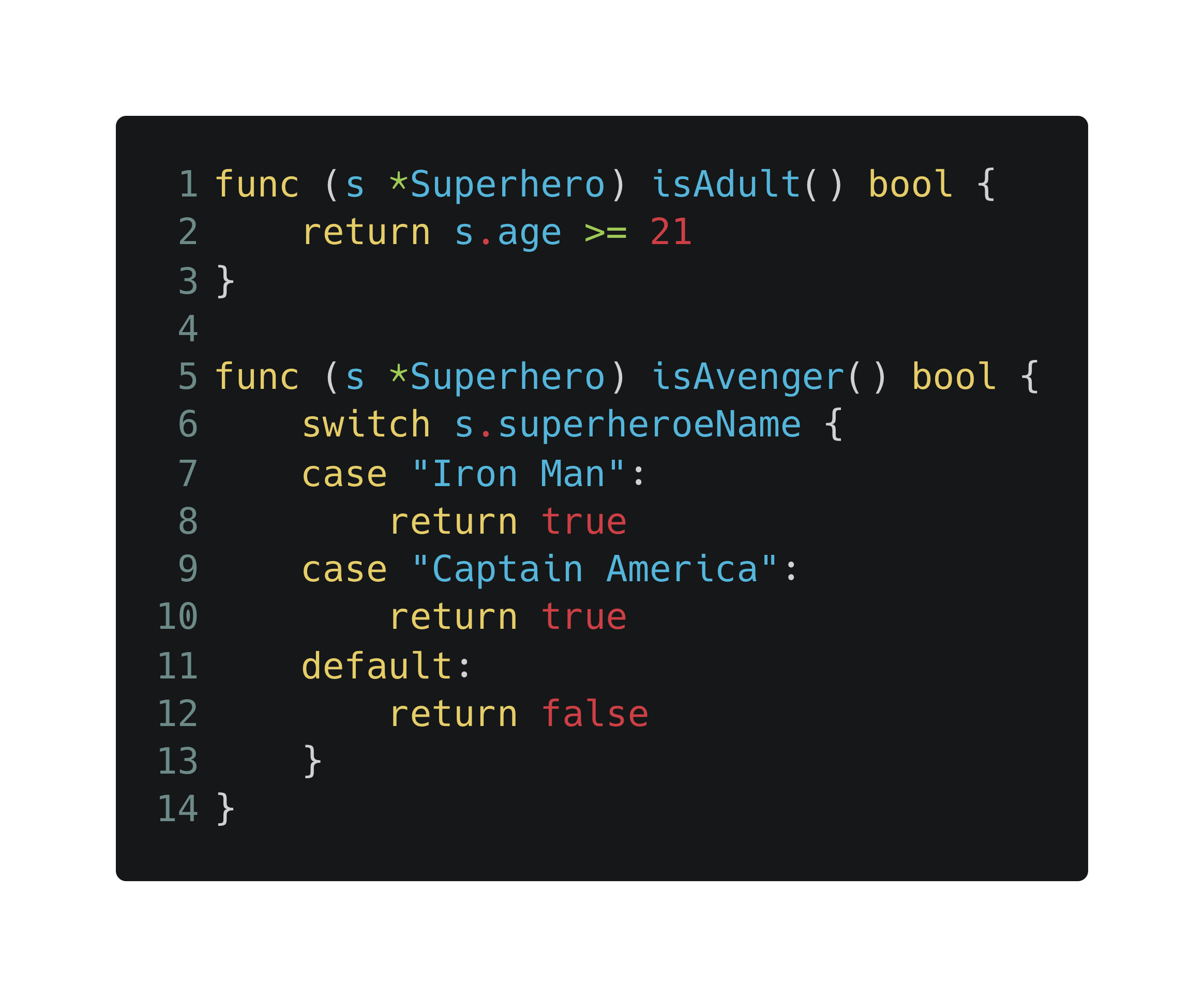
Do we double-click each function to generate their tests? No! You can create tests for every function in a source file by opening VSC's Command Palette and selecting the "Go: Generate Unit Tests For File" option.

Doing so will add the following code to the existing test file:
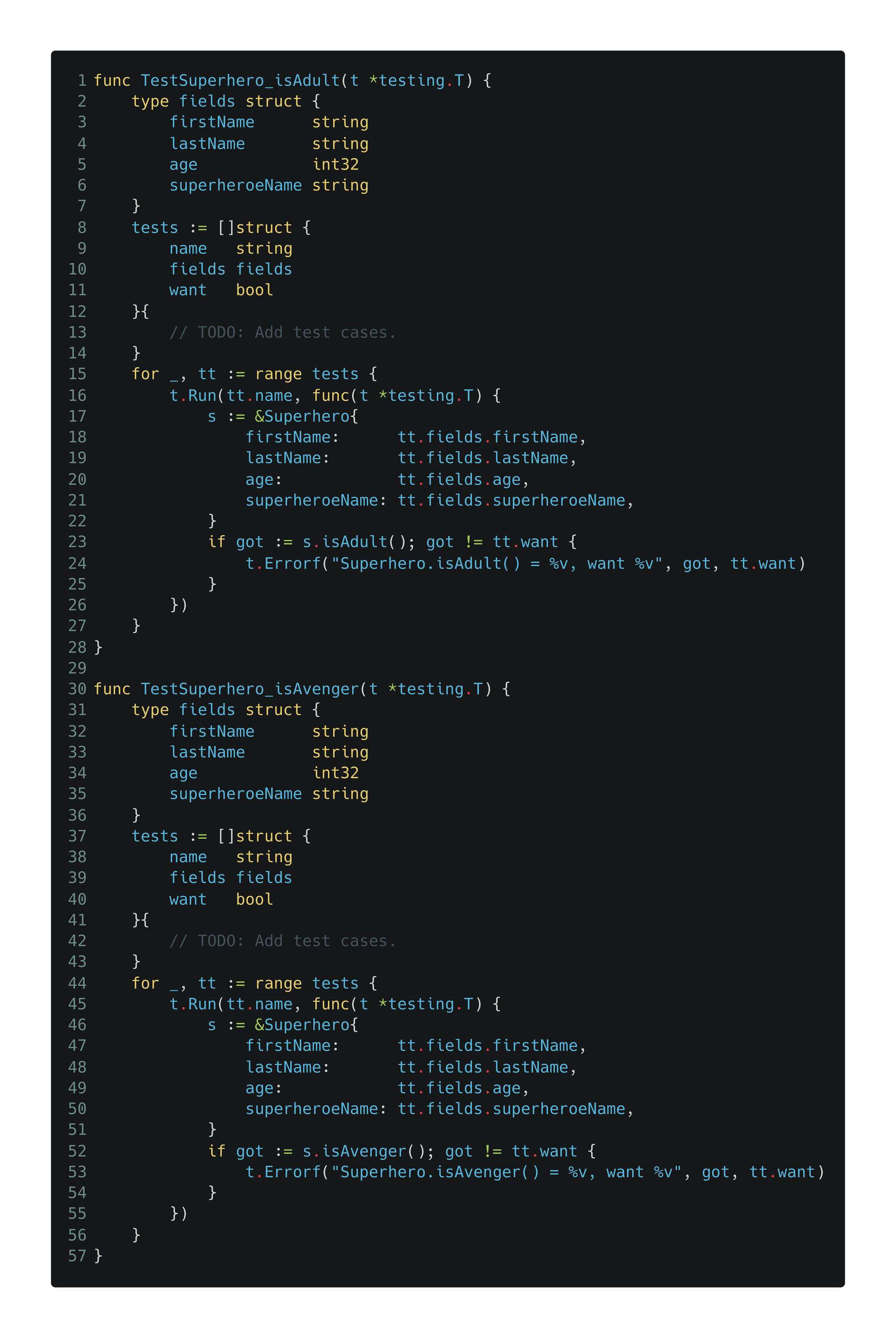
From this point, it's up to you to finish your tests and, if needed, modify the boilerplate code to suit your requirements. Happy testing!